Selecting the right programming language is a pivotal decision for any development project. Whether you work within the Android ecosystem or in broader software development, you’ve likely debated between the modern, dynamic Kotlin and the years-proven Java. Both offer unique advantages, catering to different types of developers and projects. In this post, we’ll dive deep into their features, compare their strengths, and help you decide which language best suits your goals.
How Did We Get Here? A Quick History
Java
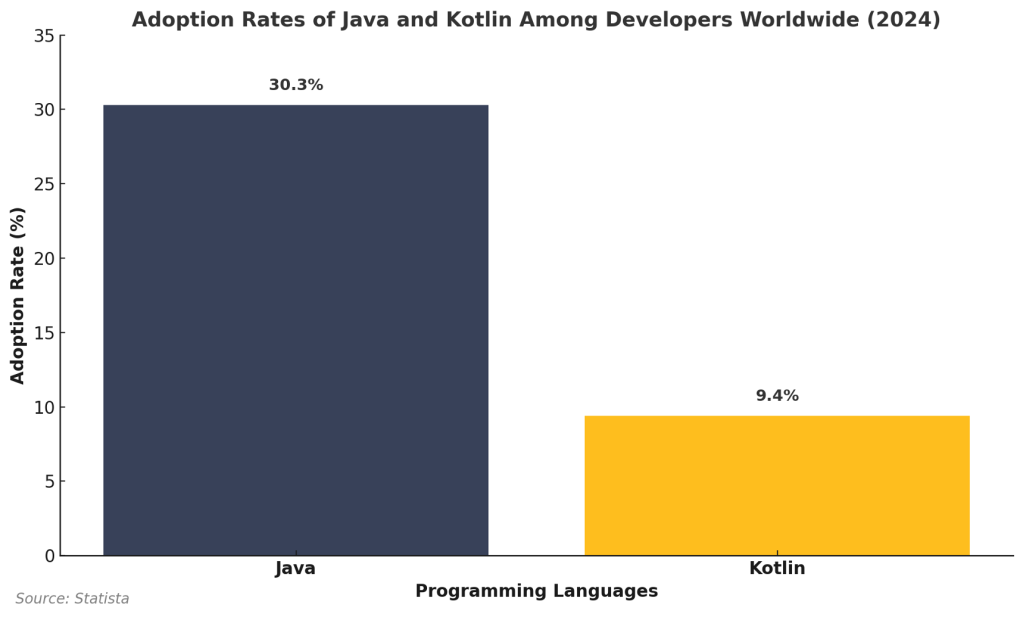
Released in 1995 by Sun Microsystems, Java revolutionized software development with its “write once, run anywhere” philosophy. It powers enterprise systems, Android apps, and large-scale backend solutions, supported by a massive ecosystem of tools and frameworks. Java is still highly relevant today with backend frameworks like Spring and Quarkus, proving its versatility in both legacy and modern systems.
Kotlin
JetBrains created Kotlin in 2011 and in 2017 it gained broader recognition when Google named it a preferred language for Android development. Designed to address Java’s shortcomings, Kotlin combines object-oriented and functional programming paradigms, offering modern features, concise syntax, and developer-friendly tools. Kotlin is primarily known for Android development, however, its applications are expanding into backend systems, web development, and even data science.
The Features That Set Them Apart
Both Kotlin and Java operate on the JVM and offer interoperability, but their design philosophies differ significantly. Here’s a comparison of their key features:
Feature | Java | Kotlin |
---|---|---|
Paradigm | Primarily object-oriented | Supports both object-oriented and functional programming |
Null Safety | Allows null values by default, leading to potential NullPointerExceptions (NPEs) | Built-in safeguards prevent most null errors at compile time |
Syntax | Verbose, requiring more code | Concise and expressive, with reduced boilerplate |
Casting | Manual type checks and casts are required. | Smart casts handle redundant type checks automatically |
Exceptions | Requires catching or declaring exceptions | No need to catch or declare exceptions, offering flexibility |
Data Classes | Developers must manually create constructors, getters, setters, and other methods | A single data keyword generates all necessary methods |
Concurrency | Multiple threads are supported but require complex handling | Simplifies concurrency with coroutines |
Performance | Optimized for stability and scalability | Slight trade-offs in speed for added features |
Interoperability | Works seamlessly with Java libraries | Fully interoperable with existing Java code |
Object-Oriented vs. Functional Programming
Java is primarily an object-oriented programming language, which provides a solid foundation for building large-scale, maintainable systems. However, it can result in verbose and repetitive code. Kotlin, on the other hand, supports both object-oriented and functional programming paradigms. This flexibility allows developers to write cleaner, more expressive code and enables advanced features like type-safe builders for constructing complex data structures.
Null Safety
NullPointerExceptions (NPEs) are a notorious pain point for Java developers. In Java, any variable can be assigned a null value by default, which can lead to runtime crashes if not handled properly. For example:
Java Example
javaCopy codeString name = "Curotec";
if (name != null) {
System.out.println(name.length());
}
Kotlin takes a safer approach by preventing null assignments unless explicitly declared with a nullable type (?
). This eliminates most NPEs at compile time:
Kotlin Example
kotlinCopy codeval name: String? = "Curotec"
println(name?.length)
With Kotlin, the code is not only safer but also cleaner and less prone to error.
👋 Do you need expert help with your Java or Kotlin project?
Casting
In Java, developers must explicitly check and cast variable types, which can result in verbose code:
Java Example
javaCopy codeif (obj instanceof String) {
String str = (String) obj;
System.out.println(str.length());
}
Kotlin simplifies this process with smart casts, which automatically handle type checks and casts:
Kotlin Example
kotlinCopy codeif (obj is String) {
println(obj.length)
}
This feature reduces boilerplate and streamlines development
Exceptions
Java enforces a strict error-handling policy, requiring developers to catch or declare exceptions. While this ensures robust and reliable code, it can slow down the development process:
Java Example
javaCopy codetry {
riskyMethod();
} catch (IOException e) {
e.printStackTrace();
}
Kotlin, on the other hand, does not require exception handling, offering greater flexibility. While this can save time, it’s up to developers to ensure proper error manageme
Data Classes
Creating data classes in Java can be a repetitive process involving constructors, getters, setters, and additional methods:
Java Example
javaCopy codepublic class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
// equals(), hashCode(), and toString() methods here
}
Kotlin simplifies this with the data
keyword, generating all required methods automatically:
Kotlin Example
kotlinCopy codedata class Person(val name: String, val age: Int)
Concurrency
Both Kotlin and Java support multi-threading for handling background operations. However, Java’s approach requires significant complexity to avoid thread interference. Kotlin simplifies concurrency with coroutines, which allow developers to write asynchronous code more easily:
Kotlin Example
kotlinCopy codelaunch {
val result = fetchData()
println(result)
}
This makes Kotlin a better choice for projects requiring extensive concurrency, such as Android apps
Community and Ecosystem Support
Java has one of the largest and most active developer communities, with an unmatched ecosystem of libraries and frameworks like Spring and Quarkus for backend development. Kotlin, while newer, benefits from strong backing by JetBrains and Google, particularly in Android development. Its growing library support and documentation make it increasingly viable for a wide range of proj
What Lies Ahead?
Java’s continued evolution, with features like records and pattern matching, ensures its relevance for modern development. Frameworks like Spring continue to dominate in backend systems, making Java a strong contender for new projects, not just legacy ones.
Kotlin, meanwhile, is expanding beyond Android, proving its versatility in backend systems, web development, and data science. Its growing popularity suggests it will continue to be a key player in modern development.
Making Your Decision
The right choice depends on your project’s needs and your team’s expertise. Here are some guidelines:
When to Choose Java
- Large, enterprise-grade systems
- Backend development with frameworks like Spring or Quarkus
- Teams already experienced in Java
When to Choose Kotlin
- Android app development (Google’s preferred language)
- Projects benefiting from concise syntax and modern features
- Agile teams focused on rapid development
For backend systems, the decision often comes down to team familiarity, as both Java and Kotlin handle most use cases effectively.
Closing Thoughts
Kotlin and Java aren’t competitors—they’re collaborators. Their seamless interoperability allows teams to transition gradually, leveraging the best of both worlds. Whether you stick with Java’s stability or embrace Kotlin’s modernity, both languages are powerful tools for building scalable, reliable software.
Ready to start your next project? Curotec’s expert developers can help you make the right choice and bring your vision to life. Get in touch to discuss how we can help!